Using Imgui to Make Tools
ImGui, short for Immediate Mode GUI, is a bloat-free graphical user interface library for C++. It’s well suited for tooling and real-time applications where ease of use, code efficiency, and speed of development are keys. ImGui’s immediate mode paradigm means that your UI is rendered and managed in real-time, making it responsive and adaptable to changes.
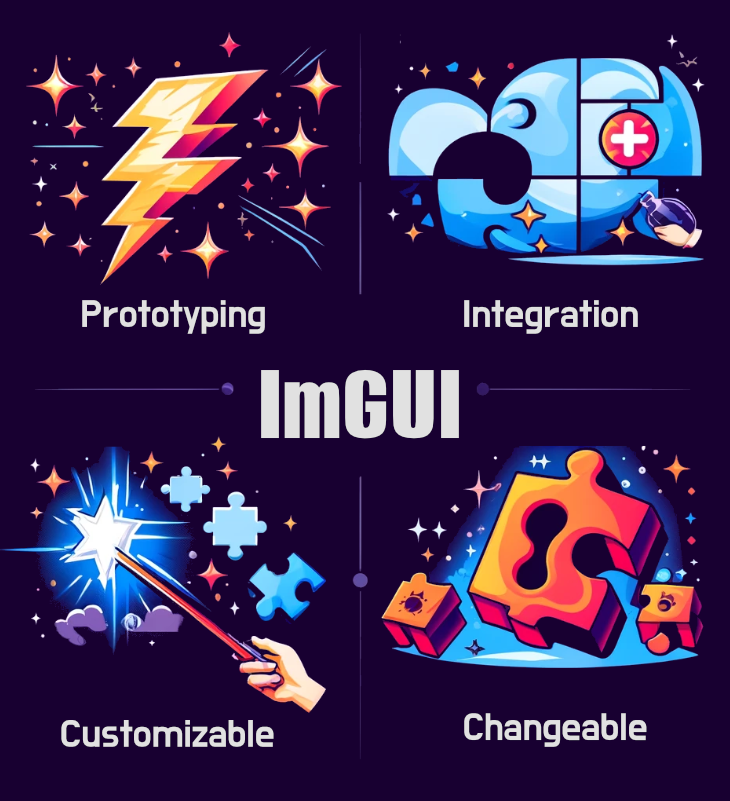
Easy to Use
ImGui is especially well-suited for developing tools due to its simplicity and flexibility. You can quickly prototype and iterate over your GUI, making it easy to add or modify UI elements as your tool is updated.
Integration with Other Libraries
ImGui can be easily integrated with a wide range of libraries, which is importent for most applications that often depend on external libraries for data collection, processing, and visualization. For instance, ImGui can be used alongside:
OpenGL or DirectX for rendering.
Data processing libraries like OpenCV for image data, librosa for audio data, or custom libraries for handling specific types of sensors or inputs. The ImGui repository itself provides examples of integrations with these rendering libraries, which can serve as a solid starting point for your tool.
Customizable Widgets
While ImGui comes with a variety of built-in widgets, one of its strengths is the ability to create custom widgets. This is particularly beneficial for a data acquisition tool where you might need bespoke controls or visualization widgets to display the acquired data effectively. Here’s a simplified example of how you might extend ImGui with a custom widget:
void CustomDataVisualizationWidget(const char* label, const MyData& data) {
// Use ImGui primitives to draw your custom widget, for example:
ImGui::Begin(label);
// Custom rendering code here, utilizing ImGui::DrawList API
// for drawing custom shapes, lines, or using custom colors
ImGui::End();
}
This flexibility allows you to tailor the UI to your application’s needs.
Leveraging C++17 Features
ImGui and C++17 are a great match. C++17 features like std::optional, structured bindings, and std::variant can simplify your code, making it more readable and maintainable.
Conclusion
ImGui is an excellent choice for developing tools in C++. It offers the speed, flexibility, and ease of use necessary for rapid development and iteration. Combined with C++17 and the ability to integrate with a wide range of libraries, ImGui empowers developers to build sophisticated, user-friendly tools tailored to their specific data needs. Whether you’re collecting sensor data, processing images, or capturing streams of information, ImGui can help you build a tool that is both powerful and easy to use.