Smart Pointer Essentials in C++11
From C++11, smart pointers such as std::unique_ptr and std::shared_ptr were introduced to replace older techniques like raw pointers, which require manual management of memory allocation and deallocation.
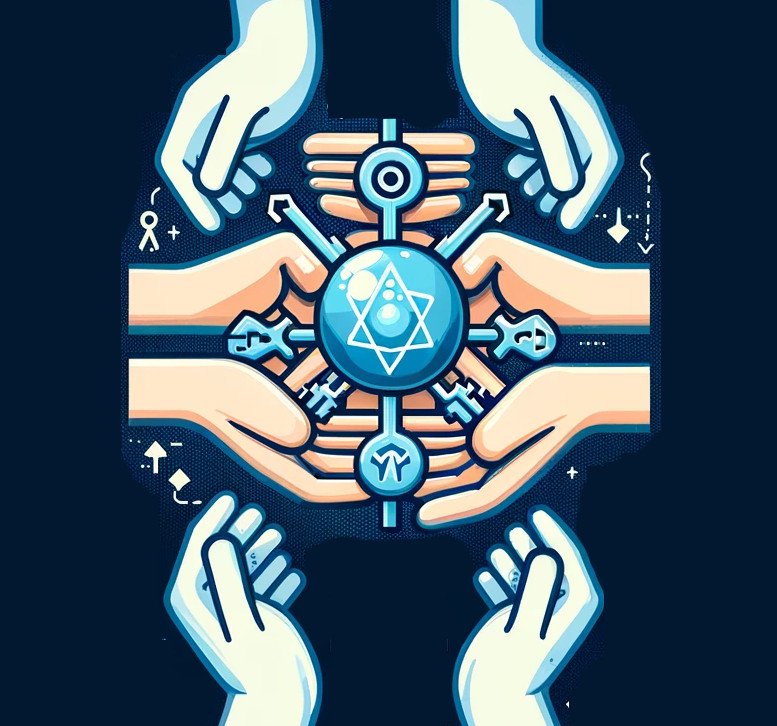
Smart pointers help manage the lifetime of dynamically allocated objects in a more robust and exception-safe manner. Here’s why using std::unique_ptr and std::shared_ptr is recommended:
1. std::unique_ptr
std::unique_ptr is a smart pointer that owns and manages another object through a pointer and disposes of that object when the std::unique_ptr goes out of scope. Unlike raw pointers, std::unique_ptr automatically releases the memory it points to when it is no longer needed, without needing explicit deletion.
Advantages of std::unique_ptr:
- Ownership Semantics: std::unique_ptr clearly communicates the ownership of a resource. There is exactly one owner of the underlying pointer, which makes the management and intent of resources clear.
- Memory Safety: It helps prevent memory leaks by ensuring that the memory is automatically deallocated when no longer needed. This is particularly useful in the presence of exceptions, where an early exit might skip manual deallocation.
- Performance: std::unique_ptr has almost no overhead over raw pointers in terms of performance. It provides automatic memory management without additional cost in most cases.
- Transfer of Ownership: Ownership of the pointer can be transferred to another std::unique_ptr using move semantics, which is efficient and safe.
2. std::shared_ptr
std::shared_ptr manages the lifetime of an object through reference counting. Multiple std::shared_ptr instances can share the same object. The object is destroyed and its memory deallocated when the last std::shared_ptr owning the object is destroyed or reset.
Advantages of std::shared_ptr:
- Shared Ownership: Useful when an object needs to be accessed by multiple parts of a program without necessarily imposing strict ownership rules.
- Automatic Lifetime Management: Like std::unique_ptr, std::shared_ptr automatically manages the memory of the object it owns, which helps prevent memory leaks.
- Ease of Use: Managing the reference count is automatic, so the programmer doesn’t need to explicitly write code to keep track of how many pointers are referencing an object.
- Cycle Detection: std::shared_ptr can be combined with std::weak_ptr to create weak references and avoid memory leaks due to reference cycles, although detecting and managing cycles requires additional care.
When to Use Each
Use std::unique_ptr when there is a single owner for the dynamically allocated object. This is often the case when you are implementing simple scoped ownership semantics without the need for sharing an object.
Use std::shared_ptr when objects need to be shared across several parts of an application, or when you need to implement more complex structures like trees or linked structures that are accessed from multiple places.
Problem with Circular References
Imagine two objects, A and B, each with a std::shared_ptr pointing to the other. This creates a loop: A owns B and B owns A. Even if every other part of your program stops using A and B, they won’t be deleted because they still own each other. This is called a “circular reference”, and it leads to memory leaks because the memory used by A and B is never released.
Role of std::weak_ptr
std::weak_ptr is designed to break these circular references. It’s a type of smart pointer that references an object managed by std::shared_ptr, but it doesn’t contribute to the reference count. This means a std::weak_ptr allows you to access an object that might be owned by one or more std::shared_ptrs, but it won’t keep it alive by itself.
When to Use std::weak_ptr with std::shared_ptr
You should use std::weak_ptr when:
- You want to reference an object that is owned by std::shared_ptrs, but you don’t need to extend its lifetime. This is common in cache implementations where objects can expire independently of their usage in the program.
- You need to break a circular reference to prevent memory leaks. For example, if you have two classes, Parent and Child, where Parent has a std::shared_ptr to Child and Child has a std::shared_ptr back to Parent, replace the Child’s std::shared_ptr to Parent with a std::weak_ptr. This way, Child can access Parent, but it doesn’t keep it alive.
Conclusion
Overall, the introduction of smart pointers in C++11 has greatly simplified resource management in C++, reducing the common errors associated with dynamic memory management, such as leaks, dangling pointers, and double deletions. They are a critical tool in modern C++ programming, promoting safer and more maintainable code.