Introduction to Data Driven Programming Using ECS
Entity Component System (ECS) architecture and traditional Object-Oriented Programming (OOP) offer different approaches to organizing code and data during development. Understanding these differences can help you decide which is more suitable for your application. Here’s a breakdown of their key differences, focusing on performance and flexibility:
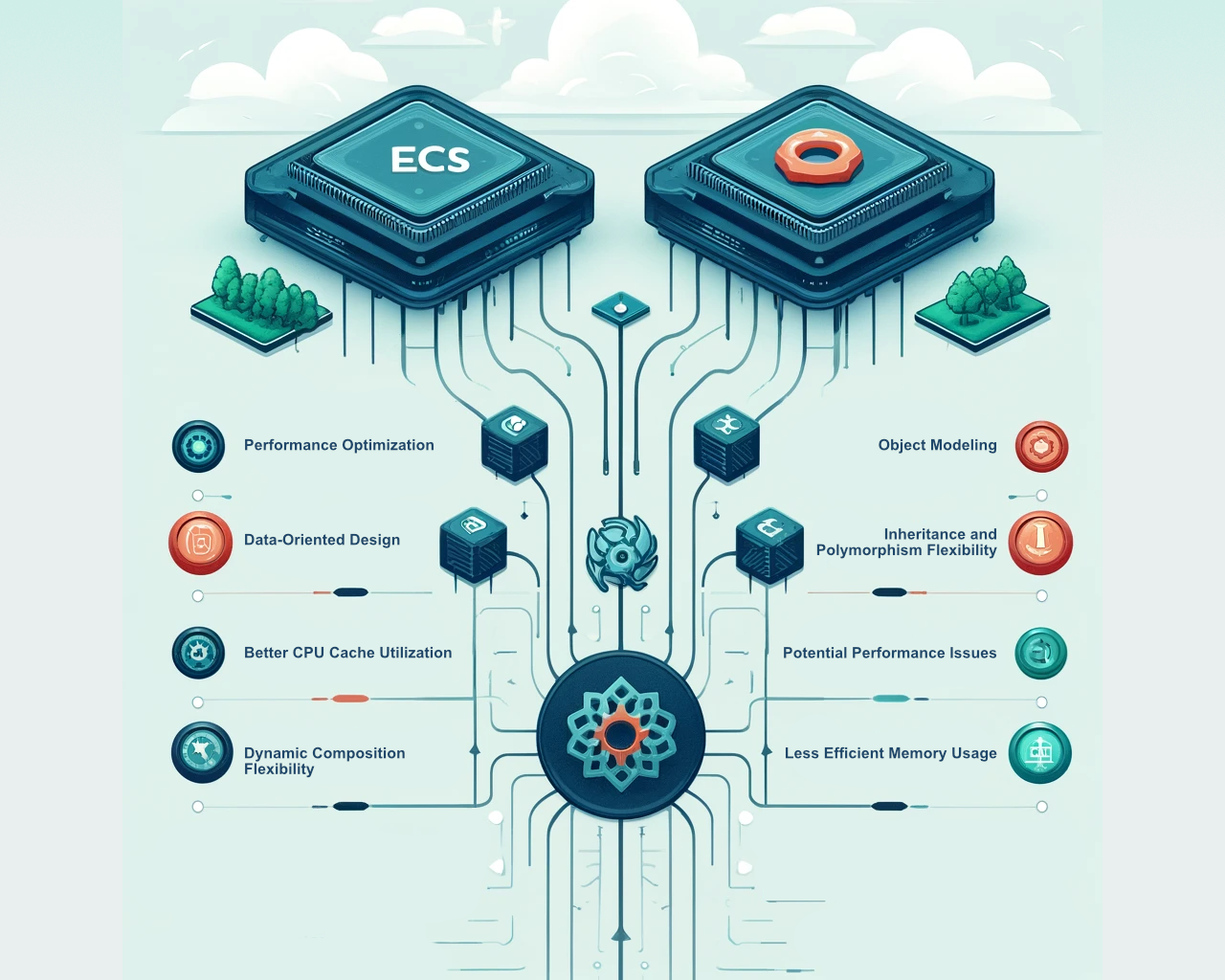
Basics:
OOP:
- In OOP, objects are the primary way to model behavior and state. An object combines data and methods that operate on that data.
- A common pattern in game development is the use of inheritance to create hierarchies of classes that share behavior and data.
ECS:
- ECS is a compositional design pattern, where you break down objects into three main components: Entities, Components, and Systems.
- Entities are unique identifiers.
- Components are plain data structures that represent various aspects of an entity (position, velocity, etc.).
- Systems contain the logic that operates on entities with specific component combinations.
Performance:
OOP:
- OOP can lead to less efficient memory usage and cache performance, especially if a game’s inheritance hierarchies become deep and complex. This is due to the fact that related data might not be stored closely in memory, leading to cache misses.
- Dynamic dispatch (polymorphism) can further impact performance, as it introduces runtime overhead.
ECS:
- ECS tends to offer better performance, particularly in systems where many objects must be processed every frame. This is because it encourages data-oriented design, which can optimize memory layout for iteration speed. By storing components contiguously in memory and processing them in systems, ECS can take advantage of CPU cache more effectively, reducing cache misses.
- ECS architecture can lead to more predictable performance as systems operate on batches of components.
Flexibility:
OOP:
- OOP offers a high degree of flexibility through inheritance and polymorphism, allowing for easy implementation of complex behaviors.
- However, deep inheritance trees can become hard to manage and extend, leading to tight coupling between objects and their behaviors.
ECS:
- ECS provides a different kind of flexibility by decoupling data from logic. This allows for more dynamic composition of entity behaviors at runtime.
- Adding or modifying behaviors in ECS is often as simple as adding or removing components from entities, making it easier to iterate on game design without touching the underlying system logic.
- ECS can also facilitate easier collaboration between programmers and designers, as system logic and entity composition can be worked on independently.
Conclusion for Your Application:
- If your application or game involves a lot of dynamic interactions, entities with varying sets of behaviors, or you’re aiming for high performance, ECS could be beneficial.
- ECS can simplify managing the diverse elements of an application or game (e.g., characters, items, platforms) by treating them as compositions of components, which can be more scalable and easier to debug than deep OOP hierarchies.
- However, it requires a shift in thinking and design strategy, so there might be a learning curve involved.
Ultimately, the choice between ECS and OOP for your game depends on your specific needs, the scale of your project, and your comfort level with these paradigms. ECS offers significant advantages in terms of performance and flexibility, which can be particularly beneficial in game development where both aspects are critical.
April 9, 2024 ∙